In today’s interconnected world, your application’s success often hinges on its ability to speak
the language of its users—literally. Internationalization (i18n)
is no longer a luxury; it’s a
necessity for any backend developer aiming to create truly global applications. By mastering i18n,
you’re not just translating words; you’re opening doors to new markets, enhancing user experiences,
and future-proofing your code. This guide will empower you with the knowledge and tools to
transform your backend into a multilingual powerhouse, ensuring your app feels native to users
across the globe. Ready to take your backend beyond borders? Let’s dive in!
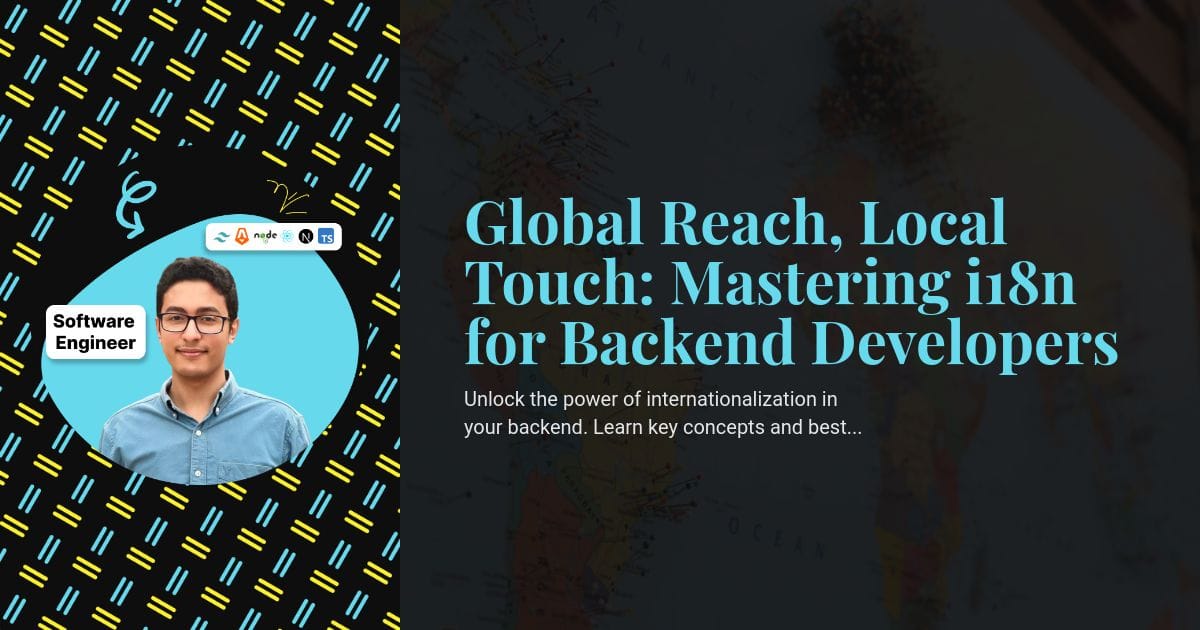
Contents
- Why Internationalization Matters
- Key Concepts of i18n
- Implementing i18n in Your Backend
- Best Practices for i18n Development
- Conclusion
Why Internationalization Matters
Understanding the importance of internationalization is crucial for any developer looking to create applications with global appeal. Let’s delve into the key benefits and challenges of implementing i18n in your backend.
Benefits of i18n
Expanded Market Reach
: By supporting multiple languages, you can attract users from diverse linguistic backgrounds, significantly expanding your potential user base. This is particularly important in today’s global digital economy, where users expect applications to cater to their language preferences.- Increases potential user base exponentially
- Opens up new markets and revenue streams
- Enhances global brand presence and recognition
Improved User Experience
: Users feel more comfortable and engaged when interacting with an application in their native language. This leads to higher user satisfaction, increased engagement, and improved retention rates.- Reduces cognitive load for non-native speakers
- Increases user confidence and trust in the application
- Facilitates more intuitive and natural interactions
Compliance with Local Regulations
: Many regions require applications to be available in local languages, making i18n crucial for legal compliance. This is especially important for applications dealing with sensitive information or operating in regulated industries.- Ensures adherence to local language laws
- Facilitates easier entry into new markets
- Demonstrates commitment to local users and markets
Challenges of Multilingual Applications
While the benefits are clear, implementing i18n comes with its own set of challenges that developers must navigate:
Complex Setup
: Integrating i18n into an existing application can be complex and time-consuming. It often requires restructuring parts of your codebase and carefully planning how to handle dynamic content.- Requires careful planning and architecture
- May necessitate refactoring existing code
- Involves setting up new build processes and tooling
Performance Considerations
: Poorly implemented i18n can lead to performance issues, especially with large translation files. Developers need to be mindful of how translations are loaded and cached to ensure optimal application performance.- Large translation files can impact load times
- Dynamic loading of translations may affect runtime performance
- Caching strategies need to be implemented carefully
Context-Sensitive Translations
: Some phrases may have different meanings in different contexts, requiring careful translation management. This can be particularly challenging for languages with complex grammar rules or cultural nuances.- Requires deep understanding of target languages and cultures
- May necessitate working closely with professional translators
- Demands careful organization of translation keys and contexts
Right-to-Left (RTL) Languages
: Supporting languages like Arabic requires additional considerations for layout and design. This often goes beyond mere translation and can impact the entire user interface.- Requires adjustments to UI layouts and components
- May necessitate changes to CSS and design principles
- Involves testing and QA processes specific to RTL languages
Key Concepts of i18n
To effectively implement internationalization, it’s crucial to understand the fundamental concepts and terminology associated with i18n. Let’s explore these key ideas that form the foundation of multilingual application development.
Internationalization vs. Localization
Understanding the difference between internationalization and localization is crucial for effective global application development:
-
Internationalization (i18n)
: The process of designing and developing an application so that it can be adapted to various languages and regions without engineering changes. This involves:- Separating user-facing strings from code
- Using Unicode for character encoding
- Designing flexible layouts that can accommodate different text lengths
- Implementing date, time, and number formatting that can adapt to different locales
-
Localization (L10n)
: The process of adapting internationalized software for a specific region or language by translating text and adding locale-specific components. This includes:- Translating user interface elements
- Adapting graphics and icons to cultural norms
- Modifying content to suit local preferences
- Ensuring compliance with local regulations and standards
Common i18n Terms
Familiarizing yourself with these terms will help you navigate the world of internationalization more effectively:
Locale
: A set of parameters that defines the user’s language, region, and any special variant preferences. It typically includes:- Language code (e.g., ‘en’ for English)
- Country or region code (e.g., ‘US’ for United States)
- Sometimes, script code or other variants (e.g., ‘zh-Hans’ for Simplified Chinese)
Translation Key
: A unique identifier used to look up translated text. Best practices for translation keys include:- Using descriptive, context-based names
- Organizing keys hierarchically
- Avoiding hardcoded strings in your code
Fallback Language
: The default language used when a translation is not available in the requested language. Considerations for fallback languages:- Usually set to the application’s primary development language
- Can be configured on a per-key basis in some i18n libraries
- Helps ensure that no part of the UI is left untranslated
Implementing i18n in Your Backend
Now that we’ve covered the foundational concepts, let’s dive into the practical aspects of implementing internationalization in your backend application. We’ll use a Node.js environment with Express and the i18next library as our example.
Choosing an i18n Library
For our Node.js backend, we’ll use the popular i18next 🔗 library along with its Express middleware. This combination provides a robust and flexible i18n solution. Here’s why i18next is a great choice:
- Mature and well-maintained library with a large community
- Supports both backend and frontend internationalization
- Offers a wide range of plugins and integrations
- Provides TypeScript support for type-safe translations
Setting Up Your Project
Let’s look at the project structure and key files:
project/
├── package.json
├── src/
│ ├── i18n.ts
│ ├── index.ts
│ └── locales/
│ ├── en.json
│ └── ar.json
└── ...
This structure allows for clear separation of concerns and easy management of translation files.
First, let’s examine our package.json
:
{
"name": "global-reach-local-touch-mastering-i18n-for-backend-developers",
"version": "1.0.0",
"dependencies": {
"express": "^4.19.2",
"i18next": "^23.14.0",
"i18next-fs-backend": "^2.3.2",
"i18next-http-middleware": "^3.6.0"
}
}
These dependencies provide everything we need to set up i18n in our Express application:
express
: Our web application frameworki18next
: The core internationalization libraryi18next-fs-backend
: Allows loading translations from the file systemi18next-http-middleware
: Integrates i18next with Express
Note: Be sure to install these dependencies using
npm
,pnpm
, oryarn
.
Next, let’s create our i18n.ts
file to configure i18next and set up the middleware:
import { type Request } from "express";
import i18next from "i18next";
import i18nextMiddleware from "i18next-http-middleware";
import Backend from "i18next-fs-backend";
import en from "@/locales/en.json";
import ar from "@/locales/ar.json";
i18next
.use(Backend)
.use(i18nextMiddleware.LanguageDetector)
.init({
fallbackLng: "en",
resources: {
en: { translation: en },
ar: { translation: ar },
},
});
export { i18next, i18nextMiddleware };
This file initializes i18next with our translation files and sets up the middleware to handle language detection.
Creating Translation Files
We’ve created two translation files: en.json
for English and ar.json
for Arabic.
Here’s an example of the English file:
{
"welcome": "Welcome",
"blog": {
"title": "Global Reach, Local Touch: Mastering i18n for Backend Developers",
"description": "Unlock the power of internationalization in your backend. Learn key concepts and best practices for implementing i18n, enabling your application to reach a global audience while providing a localized experience."
}
}
This structure allows for easy organization of translations and supports nested keys for more complex hierarchies. When creating translation files:
- Use consistent naming conventions across all language files
- Keep translations atomic and context-specific
- Consider using a translation management system for larger projects
Generating Translation Keys Automatically
In our i18n.ts
file, we’ve implemented a clever TypeScript solution to generate
translation keys automatically:
type NestedKeyOf<T> = T extends object
? {
[K in keyof T]: K extends string ? (T[K] extends object ? `${K}.${NestedKeyOf<T[K]>}` : K) : never;
}[keyof T]
: never;
const keys = { ...en } as const;
type TranslationKeys = NestedKeyOf<typeof keys>;
This type definition offers several advantages:
- Provides autocompletion for translation keys in your IDE
- Catches typos and invalid keys at compile-time
- Makes refactoring easier and safer
- Ensures consistency between your code and translation files
Next, let’s create a simple translation helper function to access our translations:
function t(key: TranslationKeys, option: Request | { lang: string | undefined }) {
if ("lang" in option) {
return i18next.t(key, { lng: option.lang?.toLocaleLowerCase() });
}
return option.t(key);
}
This function abstracts the translation lookup process and handles language detection automatically.
Note:
t
is a simplified example. You can extend it to support more advanced features like placeholders, formatting, and pluralization.
By leveraging TypeScript’s type system, we can create a more robust and maintainable internationalization setup.
Add i18n Middleware to Express
Finally, let’s add the i18n middleware to our Express application:
import express from "express";
import cors from "cors";
import { i18next, i18nextMiddleware, t } from "@/i18n";
const app = express();
app.use(i18nextMiddleware.handle(i18next));
app.use(cors());
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.use(express.static("public"));
const PORT = process.env.PORT || 3000;
app.get("/", (req, res) => {
const welcome = t("welcome", req);
return res.send(welcome);
});
app.get("/blog", (req, res) => {
const lang = req.language;
const blog = {
title: t("blog.title", { lang }),
description: t("blog.description", { lang }),
};
res.json(blog);
});
app.listen(PORT, () => {
console.log(`Server running on: http://localhost:${PORT} 🚀`);
});
This code snippet demonstrates how to integrate i18n into your Express application,
Best Practices for i18n Development
Implementing i18n effectively requires more than just translating strings. Let’s explore some best practices that will help you create a scalable and maintainable internationalized application.
Organizing Translation Files
Proper organization of translation files is crucial for managing translations effectively as your application grows:
- Keep translation files small and modular:
- Split translations into logical sections or features
- Consider using multiple files for large applications
- Use a consistent naming convention for files (e.g.,
common.json
,errors.json
,features.json
)
- Use nested objects to group related translations:
- Helps maintain context and reduces key conflicts
- Makes it easier to understand the structure of your translations
- Example:
{ "user": { "profile": { "title": "Profile", "description": "User profile settings" } } }
- Consider using a translation management system for larger projects:
- Provides a centralized platform for managing translations
- Allows for easier collaboration with translators
- Often includes features like version control and in-context editing
Handling Dynamic Content
When dealing with dynamic content, use placeholders in your translations to maintain flexibility:
{
"greeting": "Hello, {{name}}!",
"itemCount": "You have {{count}} item",
"itemCount_plural": "You have {{count}} items"
}
Then, in your code:
t('greeting', { name: 'Alice' });
t('itemCount', { count: 5 });
This approach offers several benefits:
- Allows for more natural translations in different languages
- Supports pluralization rules for different locales
- Enables the use of formatting functions for numbers, dates, etc.
Remember to provide context for translators when using placeholders, especially for languages with different grammatical structures.
Conclusion
Unlock the World with i18n
Implementing internationalization in your backend is a powerful way to expand your application’s reach and improve user experience. By following the practices outlined in this guide, you’ll be well on your way to creating a truly global application. Remember these key takeaways:
- Start with a solid understanding of i18n concepts and terminology
- Choose the right tools and libraries for your tech stack
- Organize your translations effectively and use type-safe keys
- Handle dynamic content with placeholders and context
- Implement thorough testing and debugging practices
With these strategies in place, you’ll be able to create applications that speak the language of users around the world, opening up new opportunities and markets for your software.
happy coding! 🚀